Composite Models: two-zone jets#
from jetset.jet_model import Jet
from jetset.plot_sedfit import PlotSED
from jetset.model_manager import FitModel
Here I present how to implement a two-zone model using the composite models are handled by the FitModel
class.
In the next release, I will provide a flexible convenience method (and, hopefully, the possibility to have mutual components IC emission).
We setup a two-zone model for an EC scenario, using a conical jet.
Setting the two components#
from jetset.jet_model import Jet
compact_jet=Jet(name='compact_jet',beaming_expr='bulk_theta')
===> setting C threads to 12
compact_jet.add_EC_component(['EC_DT','EC_BLR'],disk_type='BB')
#we make the jet conical
compact_jet.make_conical_jet()
adding par: R_H to R
adding par: theta_open to R
==> par R is depending on ['R_H', 'theta_open'] according to expr: R =
np.tan(np.radians(theta_open))*R_H
setting R_H to 5.715026151380672e+16
#we set the proper dependencies for the EC fields
compact_jet.set_EC_dependencies()
adding par: L_Disk to R_BLR_in
==> par R_BLR_in is depending on ['L_Disk'] according to expr: R_BLR_in =
3E17*(L_Disk/1E46)**0.5
adding par: R_BLR_in to R_BLR_out
==> par R_BLR_out is depending on ['R_BLR_in'] according to expr: R_BLR_out =
R_BLR_in*1.1
adding par: L_Disk to R_DT
==> par R_DT is depending on ['L_Disk'] according to expr: R_DT =
2E19*(L_Disk/1E46)**0.5
compact_jet.show_model()
--------------------------------------------------------------------------------
model description:
--------------------------------------------------------------------------------
type: Jet
name: compact_jet
geometry: spherical
electrons distribution:
type: plc
gamma energy grid size: 201
gmin grid : 2.000000e+00
gmax grid : 1.000000e+06
normalization: True
log-values: False
ratio of cold protons to relativistic electrons: 1.000000e+00
accretion disk:
disk Type: BB
L disk: 1.000000e+45 (erg/s)
T disk: 1.000000e+05 (K)
nu peak disk: 8.171810e+15 (Hz)
radiative fields:
seed photons grid size: 100
IC emission grid size: 100
source emissivity lower bound : 1.000000e-120
spectral components:
name:Sum, state: on
name:Sum, hidden: False
name:Sync, state: self-abs
name:Sync, hidden: False
name:SSC, state: on
name:SSC, hidden: False
name:EC_DT, state: on
name:EC_DT, hidden: False
name:DT, state: on
name:DT, hidden: False
name:Disk, state: on
name:Disk, hidden: False
name:EC_BLR, state: on
name:EC_BLR, hidden: False
external fields transformation method: blob
SED info:
nu grid size jetkernel: 1000
nu size: 500
nu mix (Hz): 1.000000e+06
nu max (Hz): 1.000000e+30
flux plot lower bound : 1.000000e-30
--------------------------------------------------------------------------------
model name | name | par type | units | val | phys. bound. min | phys. bound. max | log | frozen |
---|---|---|---|---|---|---|---|---|
compact_jet | *R(D,theta_open) | region_size | cm | 5.000000e+15 | 1.000000e+03 | 1.000000e+30 | False | True |
compact_jet | R_H(M) | region_position | cm | 5.715026e+16 | 0.000000e+00 | -- | False | False |
compact_jet | B | magnetic_field | gauss | 1.000000e-01 | 0.000000e+00 | -- | False | False |
compact_jet | NH_cold_to_rel_e | cold_p_to_rel_e_ratio | 1.000000e+00 | 0.000000e+00 | -- | False | True | |
compact_jet | theta | jet-viewing-angle | deg | 1.000000e-01 | 0.000000e+00 | 9.000000e+01 | False | False |
compact_jet | BulkFactor | jet-bulk-factor | lorentz-factor* | 1.000000e+01 | 1.000000e+00 | 1.000000e+05 | False | False |
compact_jet | z_cosm | redshift | 1.000000e-01 | 0.000000e+00 | -- | False | False | |
compact_jet | gmin | low-energy-cut-off | lorentz-factor* | 2.000000e+00 | 1.000000e+00 | 1.000000e+09 | False | False |
compact_jet | gmax | high-energy-cut-off | lorentz-factor* | 1.000000e+06 | 1.000000e+00 | 1.000000e+15 | False | False |
compact_jet | N | emitters_density | 1 / cm3 | 1.000000e+02 | 0.000000e+00 | -- | False | False |
compact_jet | gamma_cut | turn-over-energy | lorentz-factor* | 1.000000e+04 | 1.000000e+00 | 1.000000e+09 | False | False |
compact_jet | p | LE_spectral_slope | 2.000000e+00 | -1.000000e+01 | 1.000000e+01 | False | False | |
compact_jet | T_DT | DT | K | 1.000000e+02 | 0.000000e+00 | -- | False | False |
compact_jet | *R_DT(D,L_Disk) | DT | cm | 5.000000e+18 | 0.000000e+00 | -- | False | True |
compact_jet | tau_DT | DT | 1.000000e-01 | 0.000000e+00 | 1.000000e+00 | False | False | |
compact_jet | tau_BLR | BLR | 1.000000e-01 | 0.000000e+00 | 1.000000e+00 | False | False | |
compact_jet | *R_BLR_in(D,L_Disk) | BLR | cm | 1.000000e+18 | 0.000000e+00 | -- | False | True |
compact_jet | *R_BLR_out(D,R_BLR_in) | BLR | cm | 2.000000e+18 | 0.000000e+00 | -- | False | True |
compact_jet | L_Disk(M) | Disk | erg / s | 1.000000e+45 | 0.000000e+00 | -- | False | False |
compact_jet | T_Disk | Disk | K | 1.000000e+05 | 0.000000e+00 | -- | False | False |
compact_jet | theta_open(M) | user_defined | deg | 5.000000e+00 | 1.000000e+00 | 1.000000e+01 | False | False |
--------------------------------------------------------------------------------
Now we clone the compact
jet to generate the extended
region
extended_jet=compact_jet.clone()
===> setting C threads to 12
adding par: L_Disk to R_DT
==> par R_DT is depending on ['L_Disk'] according to expr: R_DT =
2E19*(L_Disk/1E46)**0.5
adding par: L_Disk to R_BLR_in
==> par R_BLR_in is depending on ['L_Disk'] according to expr: R_BLR_in =
3E17*(L_Disk/1E46)**0.5
adding par: R_BLR_in to R_BLR_out
==> par R_BLR_out is depending on ['R_BLR_in'] according to expr: R_BLR_out =
R_BLR_in*1.1
adding par: R_H to R
adding par: theta_open to R
==> par R is depending on ['R_H', 'theta_open'] according to expr: R =
np.tan(np.radians(theta_open))*R_H
Setting the composite model#
extended_jet.name='extended_jet'
from jetset.model_manager import FitModel
composite_jet=FitModel(nu_size=500, name='composite_jet')
/Users/orion/miniforge3/envs/jetset/lib/python3.10/site-packages/jetset/model_manager.py:158: UserWarning: no cosmology defined, using FlatLambdaCDM(name="Planck13", H0=67.77 km / (Mpc s), Om0=0.30712, Tcmb0=2.7255 K, Neff=3.046, m_nu=[0. 0. 0.06] eV, Ob0=0.048252)
warnings.warn(m)
composite_jet.add_component(compact_jet)
composite_jet.add_component(extended_jet)
We set the functional expression for the model composition
composite_jet.composite_expr='extended_jet+compact_jet'
composite_jet.show_pars()
model name | name | par type | units | val | phys. bound. min | phys. bound. max | log | frozen |
---|---|---|---|---|---|---|---|---|
compact_jet | *R(D,theta_open) | region_size | cm | 5.000000e+15 | 1.000000e+03 | 1.000000e+30 | False | True |
compact_jet | R_H(M) | region_position | cm | 5.715026e+16 | 0.000000e+00 | -- | False | False |
compact_jet | B | magnetic_field | gauss | 1.000000e-01 | 0.000000e+00 | -- | False | False |
compact_jet | NH_cold_to_rel_e | cold_p_to_rel_e_ratio | 1.000000e+00 | 0.000000e+00 | -- | False | True | |
compact_jet | theta | jet-viewing-angle | deg | 1.000000e-01 | 0.000000e+00 | 9.000000e+01 | False | False |
compact_jet | BulkFactor | jet-bulk-factor | lorentz-factor* | 1.000000e+01 | 1.000000e+00 | 1.000000e+05 | False | False |
compact_jet | z_cosm | redshift | 1.000000e-01 | 0.000000e+00 | -- | False | False | |
compact_jet | gmin | low-energy-cut-off | lorentz-factor* | 2.000000e+00 | 1.000000e+00 | 1.000000e+09 | False | False |
compact_jet | gmax | high-energy-cut-off | lorentz-factor* | 1.000000e+06 | 1.000000e+00 | 1.000000e+15 | False | False |
compact_jet | N | emitters_density | 1 / cm3 | 1.000000e+02 | 0.000000e+00 | -- | False | False |
compact_jet | gamma_cut | turn-over-energy | lorentz-factor* | 1.000000e+04 | 1.000000e+00 | 1.000000e+09 | False | False |
compact_jet | p | LE_spectral_slope | 2.000000e+00 | -1.000000e+01 | 1.000000e+01 | False | False | |
compact_jet | T_DT | DT | K | 1.000000e+02 | 0.000000e+00 | -- | False | False |
compact_jet | *R_DT(D,L_Disk) | DT | cm | 5.000000e+18 | 0.000000e+00 | -- | False | True |
compact_jet | tau_DT | DT | 1.000000e-01 | 0.000000e+00 | 1.000000e+00 | False | False | |
compact_jet | tau_BLR | BLR | 1.000000e-01 | 0.000000e+00 | 1.000000e+00 | False | False | |
compact_jet | *R_BLR_in(D,L_Disk) | BLR | cm | 1.000000e+18 | 0.000000e+00 | -- | False | True |
compact_jet | *R_BLR_out(D,R_BLR_in) | BLR | cm | 2.000000e+18 | 0.000000e+00 | -- | False | True |
compact_jet | L_Disk(M) | Disk | erg / s | 1.000000e+45 | 0.000000e+00 | -- | False | False |
compact_jet | T_Disk | Disk | K | 1.000000e+05 | 0.000000e+00 | -- | False | False |
compact_jet | theta_open(M) | user_defined | deg | 5.000000e+00 | 1.000000e+00 | 1.000000e+01 | False | False |
extended_jet | gmin | low-energy-cut-off | lorentz-factor* | 2.000000e+00 | 1.000000e+00 | 1.000000e+09 | False | False |
extended_jet | gmax | high-energy-cut-off | lorentz-factor* | 1.000000e+06 | 1.000000e+00 | 1.000000e+15 | False | False |
extended_jet | N | emitters_density | 1 / cm3 | 1.000000e+02 | 0.000000e+00 | -- | False | False |
extended_jet | gamma_cut | turn-over-energy | lorentz-factor* | 1.000000e+04 | 1.000000e+00 | 1.000000e+09 | False | False |
extended_jet | p | LE_spectral_slope | 2.000000e+00 | -1.000000e+01 | 1.000000e+01 | False | False | |
extended_jet | T_DT | DT | K | 1.000000e+02 | 0.000000e+00 | -- | False | False |
extended_jet | *R_DT(D,L_Disk) | DT | cm | 5.000000e+18 | 0.000000e+00 | -- | False | True |
extended_jet | tau_DT | DT | 1.000000e-01 | 0.000000e+00 | 1.000000e+00 | False | False | |
extended_jet | tau_BLR | BLR | 1.000000e-01 | 0.000000e+00 | 1.000000e+00 | False | False | |
extended_jet | *R_BLR_in(D,L_Disk) | BLR | cm | 1.000000e+18 | 0.000000e+00 | -- | False | True |
extended_jet | *R_BLR_out(D,R_BLR_in) | BLR | cm | 2.000000e+18 | 0.000000e+00 | -- | False | True |
extended_jet | L_Disk(M) | Disk | erg / s | 1.000000e+45 | 0.000000e+00 | -- | False | False |
extended_jet | T_Disk | Disk | K | 1.000000e+05 | 0.000000e+00 | -- | False | False |
extended_jet | *R(D,theta_open) | region_size | cm | 5.000000e+15 | 1.000000e+03 | 1.000000e+30 | False | True |
extended_jet | R_H(M) | region_position | cm | 5.715026e+16 | 0.000000e+00 | -- | False | False |
extended_jet | B | magnetic_field | gauss | 1.000000e-01 | 0.000000e+00 | -- | False | False |
extended_jet | NH_cold_to_rel_e | cold_p_to_rel_e_ratio | 1.000000e+00 | 0.000000e+00 | -- | False | True | |
extended_jet | theta | jet-viewing-angle | deg | 1.000000e-01 | 0.000000e+00 | 9.000000e+01 | False | False |
extended_jet | BulkFactor | jet-bulk-factor | lorentz-factor* | 1.000000e+01 | 1.000000e+00 | 1.000000e+05 | False | False |
extended_jet | z_cosm | redshift | 1.000000e-01 | 0.000000e+00 | -- | False | False | |
extended_jet | theta_open(M) | user_defined | deg | 5.000000e+00 | 1.000000e+00 | 1.000000e+01 | False | False |
Linking pars in the composite model#
Important
since we want the two-zone to share the same jet, we link all the jet-related parameters in the two model. For a pure SSC scenario, of course, you will not have the dusty tours, and the accretion disk.
linked_pars=['z_cosm','theta_open','theta','T_Disk','L_Disk','T_DT']
for par in linked_pars:
composite_jet.link_par(par_name=par,from_model='extended_jet',to_model='compact_jet')
adding par: z_cosm to z_cosm
adding par: theta_open to theta_open
adding par: theta to theta
adding par: T_Disk to T_Disk
adding par: L_Disk to L_Disk
adding par: T_DT to T_DT
Important
Spectral components which are the same (i.e. duplicated), as the DT an the Disk, must be hidden in the jet component were are linked.
#we hide the duplicated components from DT and Disk
composite_jet.extended_jet.spectral_components.DT.hidden=True
composite_jet.extended_jet.spectral_components.Disk.hidden=True
composite_jet.show_pars()
model name | name | par type | units | val | phys. bound. min | phys. bound. max | log | frozen |
---|---|---|---|---|---|---|---|---|
compact_jet | *R(D,theta_open) | region_size | cm | 5.000000e+15 | 1.000000e+03 | 1.000000e+30 | False | True |
compact_jet | R_H(M) | region_position | cm | 5.715026e+16 | 0.000000e+00 | -- | False | False |
compact_jet | B | magnetic_field | gauss | 1.000000e-01 | 0.000000e+00 | -- | False | False |
compact_jet | NH_cold_to_rel_e | cold_p_to_rel_e_ratio | 1.000000e+00 | 0.000000e+00 | -- | False | True | |
compact_jet | theta(M) | jet-viewing-angle | deg | 1.000000e-01 | 0.000000e+00 | 9.000000e+01 | False | False |
compact_jet | BulkFactor | jet-bulk-factor | lorentz-factor* | 1.000000e+01 | 1.000000e+00 | 1.000000e+05 | False | False |
compact_jet | z_cosm(M) | redshift | 1.000000e-01 | 0.000000e+00 | -- | False | False | |
compact_jet | gmin | low-energy-cut-off | lorentz-factor* | 2.000000e+00 | 1.000000e+00 | 1.000000e+09 | False | False |
compact_jet | gmax | high-energy-cut-off | lorentz-factor* | 1.000000e+06 | 1.000000e+00 | 1.000000e+15 | False | False |
compact_jet | N | emitters_density | 1 / cm3 | 1.000000e+02 | 0.000000e+00 | -- | False | False |
compact_jet | gamma_cut | turn-over-energy | lorentz-factor* | 1.000000e+04 | 1.000000e+00 | 1.000000e+09 | False | False |
compact_jet | p | LE_spectral_slope | 2.000000e+00 | -1.000000e+01 | 1.000000e+01 | False | False | |
compact_jet | T_DT(M) | DT | K | 1.000000e+02 | 0.000000e+00 | -- | False | False |
compact_jet | *R_DT(D,L_Disk) | DT | cm | 6.324555e+18 | 0.000000e+00 | -- | False | True |
compact_jet | tau_DT | DT | 1.000000e-01 | 0.000000e+00 | 1.000000e+00 | False | False | |
compact_jet | tau_BLR | BLR | 1.000000e-01 | 0.000000e+00 | 1.000000e+00 | False | False | |
compact_jet | *R_BLR_in(D,L_Disk) | BLR | cm | 9.486833e+16 | 0.000000e+00 | -- | False | True |
compact_jet | *R_BLR_out(D,R_BLR_in) | BLR | cm | 1.043552e+17 | 0.000000e+00 | -- | False | True |
compact_jet | L_Disk(M) | Disk | erg / s | 1.000000e+45 | 0.000000e+00 | -- | False | False |
compact_jet | T_Disk(M) | Disk | K | 1.000000e+05 | 0.000000e+00 | -- | False | False |
compact_jet | theta_open(M) | user_defined | deg | 5.000000e+00 | 1.000000e+00 | 1.000000e+01 | False | False |
extended_jet | gmin | low-energy-cut-off | lorentz-factor* | 2.000000e+00 | 1.000000e+00 | 1.000000e+09 | False | False |
extended_jet | gmax | high-energy-cut-off | lorentz-factor* | 1.000000e+06 | 1.000000e+00 | 1.000000e+15 | False | False |
extended_jet | N | emitters_density | 1 / cm3 | 1.000000e+02 | 0.000000e+00 | -- | False | False |
extended_jet | gamma_cut | turn-over-energy | lorentz-factor* | 1.000000e+04 | 1.000000e+00 | 1.000000e+09 | False | False |
extended_jet | p | LE_spectral_slope | 2.000000e+00 | -1.000000e+01 | 1.000000e+01 | False | False | |
extended_jet | T_DT(L,compact_jet) | DT | K | -- | -- | -- | False | True |
extended_jet | *R_DT(D,L_Disk) | DT | cm | 6.324555e+18 | 0.000000e+00 | -- | False | True |
extended_jet | tau_DT | DT | 1.000000e-01 | 0.000000e+00 | 1.000000e+00 | False | False | |
extended_jet | tau_BLR | BLR | 1.000000e-01 | 0.000000e+00 | 1.000000e+00 | False | False | |
extended_jet | *R_BLR_in(D,L_Disk) | BLR | cm | 9.486833e+16 | 0.000000e+00 | -- | False | True |
extended_jet | *R_BLR_out(D,R_BLR_in) | BLR | cm | 1.043552e+17 | 0.000000e+00 | -- | False | True |
extended_jet | L_Disk(L,compact_jet) | Disk | erg / s | -- | -- | -- | False | True |
extended_jet | T_Disk(L,compact_jet) | Disk | K | -- | -- | -- | False | True |
extended_jet | *R(D,theta_open) | region_size | cm | 5.000000e+15 | 1.000000e+03 | 1.000000e+30 | False | True |
extended_jet | R_H(M) | region_position | cm | 5.715026e+16 | 0.000000e+00 | -- | False | False |
extended_jet | B | magnetic_field | gauss | 1.000000e-01 | 0.000000e+00 | -- | False | False |
extended_jet | NH_cold_to_rel_e | cold_p_to_rel_e_ratio | 1.000000e+00 | 0.000000e+00 | -- | False | True | |
extended_jet | theta(L,compact_jet) | jet-viewing-angle | deg | -- | -- | -- | False | True |
extended_jet | BulkFactor | jet-bulk-factor | lorentz-factor* | 1.000000e+01 | 1.000000e+00 | 1.000000e+05 | False | False |
extended_jet | z_cosm(L,compact_jet) | redshift | -- | -- | -- | False | True | |
extended_jet | theta_open(L,compact_jet) | user_defined | deg | -- | -- | -- | False | True |
setting pars in the extended and compat components#
We now place the extended region at a larger scale compared to the compact one, i.e. we increase R_H
, and we scale also the particle density and the magnetic field B
.
Note
The magnetic field could be scaled automatically, setting in both the components (compact_jet
and extended_jet
) the same functional dependency as shown in Depending parameters, for the depending B field case.
composite_jet.compact_jet.parameters.R_H.val=1E18
composite_jet.extended_jet.parameters.R_H.val=5E19
composite_jet.extended_jet.parameters.gamma_cut.val=1E3
composite_jet.extended_jet.parameters.B.val=0.001
composite_jet.extended_jet.parameters.N.val=1
composite_jet.eval()
composite_jet.extended_jet.spectral_components.DT.hidden=True
composite_jet.extended_jet.spectral_components.Disk.hidden=True
composite_jet.eval()
p=composite_jet.plot_model(skip_components=False)
p.setlim(y_min=1E-16)
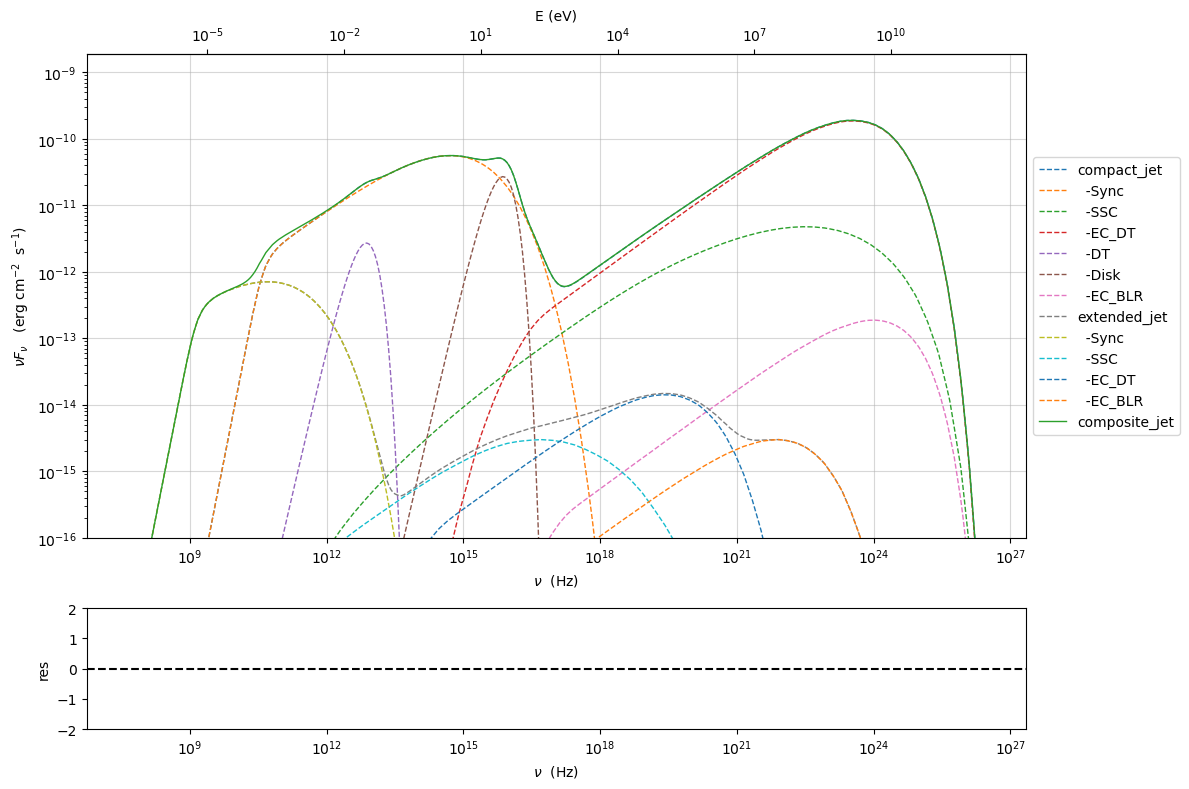